3D Transformations: Translation, Rotation, and Scaling
/ 3 min read
Introduction
I have recently developed an interest in graphics and game development. Learning these new things is always fun. I have been learning OpenGL for a while now and have found some great resources to learn from. In this blog, I will cover how to perform and combine various 3D transformations—translation, rotation, and scaling—using OpenGL and Three.js. I’ll also share some tips and code snippets that helped me along the way.
Setting Up OpenGL
To start with OpenGL, you need to set up your development environment. I primarily use VSCode for development. But also use Clion sometimes when I get it to work 😂. Below is a brief guide to set up OpenGL in VSCode.
VSCode Setup for OpenGL
YouTube video by Codeus Channel:
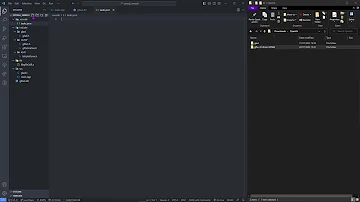
Learning
Here are some things I have learned so far.
Basic Skeleton in OpenGL
#include <iostream>
#include <glad/glad.h>
#include <GLFW/glfw3.h>
using namespace std;
int main()
{
GLFWwindow* window;
if (!glfwInit())
{
cout << "Failed to initialize GLFW" << endl;
return -1;
}
window = glfwCreateWindow(640, 480, "Hello World", 0, 0);
if (!window)
{
cout << "Failed to create window" << endl;
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
if (!gladLoadGLLoader((GLADloadproc)glfwGetProcAddress))
{
cout << "Failed to initialize GLAD" << endl;
return -1;
}
// render loop
while (!glfwWindowShouldClose(window))
{
glClearColor(1.0, 0, 0, 0);
glClear(GL_COLOR_BUFFER_BIT);
glfwSwapBuffers(window);
glfwPollEvents();
}
glfwTerminate();
}
3D Transformations
In 3D graphics, transformations are used to manipulate objects’ positions, orientations, and sizes. The three basic transformations are translation, rotation, and scaling. These transformations can be applied individually or in combination to achieve the desired effect.
1. Translation
Translation moves an object from one location to another along the x, y, and z axes.
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glTranslatef(1.0f, 0.0f, 0.0f); // Move right by 1 unit
2. Rotation
Rotation turns an object around a specified axis.
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glRotatef(45.0f, 0.0f, 0.0f, 1.0f); // Rotate 45 degrees around the z-axis
3. Scaling
Scaling changes the size of an object along the x, y, and z axes.
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glScalef(2.0f, 2.0f, 2.0f); // Scale by a factor of 2
Composite Transformations
Composite transformations combine multiple transformations into one. The order of transformations is crucial, as it affects the final outcome.
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glTranslatef(1.0f, 0.0f, 0.0f); // First, translate
glRotatef(45.0f, 0.0f, 0.0f, 1.0f); // Then, rotate
glScalef(2.0f, 2.0f, 2.0f); // Finally, scale
Three.js Transformations
Three.js is a popular JavaScript library for creating 3D graphics in the browser. It provides an easy way to apply transformations to 3D objects. Here’s how you can perform translation, rotation, and scaling using Three.js:
1. Translation
const object = new THREE.Mesh(geometry, material);
object.position.set(1, 0, 0); // Move right by 1 unit
2. Rotation
object.rotation.set(0, Math.PI / 4, 0); // Rotate 45 degrees around the y-axis
3. Scaling
object.scale.set(2, 2, 2); // Scale by a factor of 2
Composite Transformations
In Three.js, you can chain transformations together:
object.position.set(1, 0, 0); // Translate
object.rotation.set(0, Math.PI / 4, 0); // Rotate
object.scale.set(2, 2, 2); // Scale
Conclusion
Learning 3D transformations in OpenGL and Three.js has been a fascinating journey. By understanding and applying translation, rotation, and scaling, you can manipulate 3D objects to create complex scenes and animations. I hope this blog has provided you with valuable insights and practical code examples to help you in your 3D graphics development.
Signing off